Implementing OWL2 RL Profile and RDFS Inference with Python and RDFLib
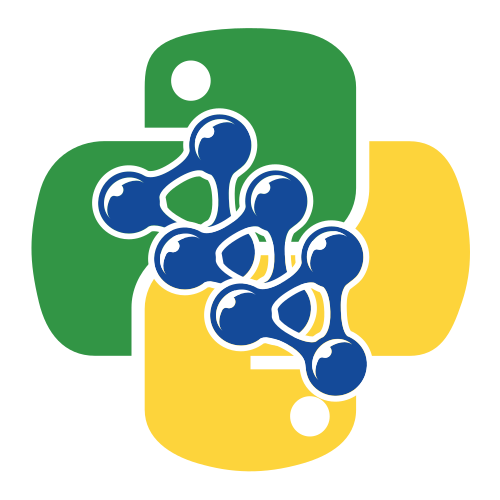
The OWL-RL library provides a simple implementation of the OWL2 RL Profile and RDFS inference on top of RDFLib, a powerful Python library for working with RDF (Resource Description Framework) data. OWL2 RL is a profile of the Web Ontology Language (OWL) that extends RDF, allowing for more expressive and automated reasoning capabilities.
In this article, we will explore three code examples that showcase the features and capabilities of OWL-RL, RDFLib, and Python. These examples will demonstrate how to perform forward chaining and transform files into RDF, using OWL-RL as the reasoning engine.
Example 1: Performing RDFS Inference
from rdflib import Graph, Namespace
from rdflib.plugin import register, Serializer
# Load RDF data into the Graph
graph = Graph()
graph.parse("data.rdf")
# Register OWL-RL reasoning plugin with RDFLib
register("owlrl", Serializer,
"rdflib.plugins.serializers.owlrl", "OWLRLSerializer")
# Perform RDFS inference
graph.serialize(destination="inferred.rdf", format="xml",
indent=True, base="http://example.org/")
In this example, we start by creating an empty Graph object from RDFLib. We then load RDF data from a file into the Graph. Next, we register the OWL-RL reasoning plugin with RDFLib using the register
function. This allows us to perform RDFS inference using OWL-RL. Finally, we serialize the inferred RDF data to a file in XML format.
Example 2: Applying OWL2 RL Profile
from rdflib import Graph, RDF, RDFS
from owlrl import OWLRL
# Load RDF data into the Graph
graph = Graph()
graph.parse("data.rdf")
# Apply OWL2 RL Profile
OWLRL.RDFSSemantics.inferClassHierarchy(graph)
OWLRL.Semantics.inferProperties(graph)
# Print the inferred triples
for s, p, o in graph:
print(s, p, o)
In this example, we again start by creating an empty Graph object from RDFLib and load RDF data into it. We then apply the OWL2 RL profile using the inferClassHierarchy
and inferProperties
methods provided by the OWLRL module. These methods perform forward chaining and infer additional triples based on RDFS and OWL2 RL rules. Finally, we print the inferred triples to the console.
Example 3: Transforming File into RDF
from rdflib import Graph, plugin
from rdflib.serializer import Serializer
# Load input file into the Graph
graph = Graph()
graph.parse("input_file.owl")
# Create the output file
output_file = open("output_file.rdf", "w")
# Serialize the Graph to RDF/XML format
plugin.register("rdfxml", Serializer, "rdflib.plugins.serializers.rdfxml", "RDFXMLSerializer")
graph.serialize(destination=output_file, format="rdfxml")
# Close the output file
output_file.close()
In this example, we create an empty Graph object and load an input file (e.g., an OWL file) into it. We then create an output file to store the transformed RDF data. Using the serialize
method provided by RDFLib, we serialize the Graph to RDF/XML format and write it to the output file. Finally, we close the output file.
These examples demonstrate the power and flexibility of RDFLib and its OWL-RL extension. With RDFLib and OWL-RL, you can easily perform RDFS inference, apply the OWL2 RL profile, and transform files into RDF. These capabilities are essential for building semantic web applications and reasoning engines.
In summary, RDFLib and OWL-RL provide a seamless integration of RDF, OWL, and RDFS within the Python ecosystem. By leveraging Python’s simplicity and RDFLib’s functionality, developers can build powerful semantic web applications and reasoning systems. So, why wait? Start exploring the possibilities of RDFLib and OWL-RL today!
For more information and detailed documentation, visit the OWL-RL documentation page: OWL-RL documentation
To stay up to date with the latest releases and updates, check out the OWL-RL repository on GitHub: OWL-RL on GitHub
Additional Information
License: W3C SOFTWARE NOTICE AND LICENSE
Author: RDFLib
Repository: OWL-RL Repository
PyPI Package: OWL-RL on PyPI
Leave a Reply